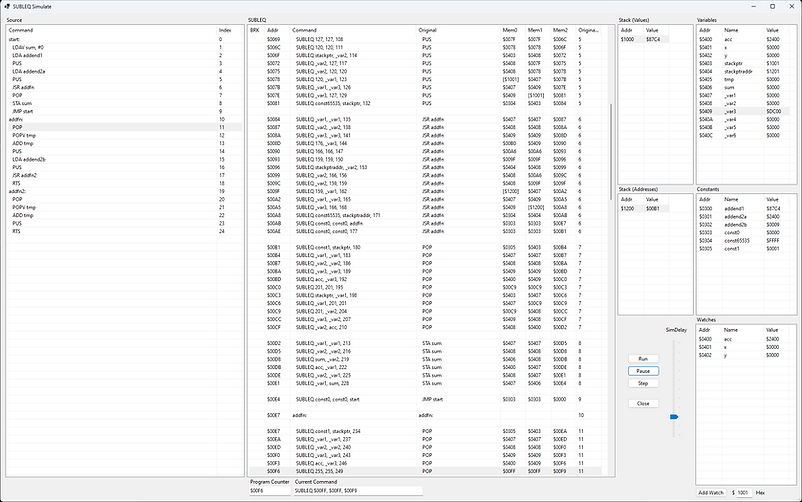
OISC Programming
Assembler
​An assembler was written in C# to help with the goal of making SUBLEQ programming accessible. Highlights of the assembler include:
-
Synthetic Registers (to act like hardware registers: Accumulator, X, Y)
-
Variables (global scope)
-
Comments
-
Constants with variable bases (decimal/hexadecimal/binary)
-
Support for ASCII characters and strings
-
Arrays
-
Address labels for jumps and subroutines
-
Built in stacks (used for subroutine program flow and passing variables to and from functions)
-
Pragma directives to the compiler - directives given to the compiler regarding the memory layout of the program, variables, stack etc.
​​​Higher level Synthetic Instructions were created from SUBLEQ instructions, and these can be used more like traditional assembler, supporting a number of addressing modes.
​
An Emulator was developed (also in C#) for testing and debugging Program Code on a PC before downloading it to the OISC.​
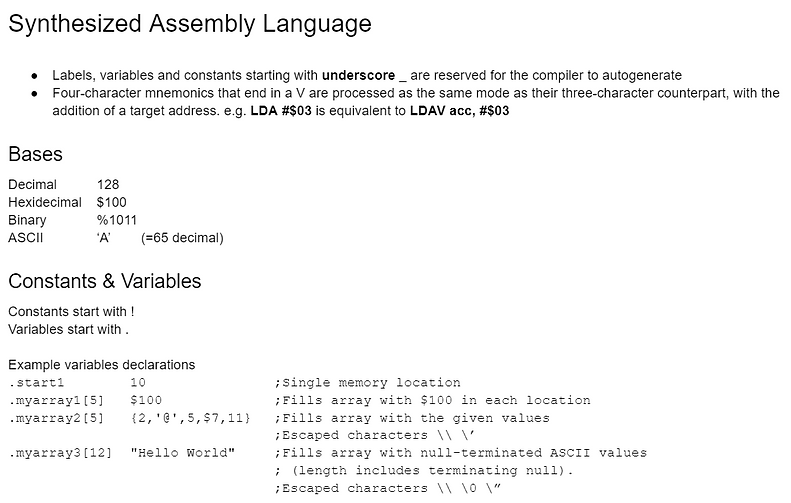

Synthetic Instructions
Around 100 synthetic instructions were created, including their different addressing modes in this count. A selection of the instructions and a list of addressing modes are given below.

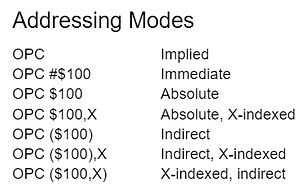
An example definition of a memory transfer instruction is given below.
-
The ARGs reference the arguments given to the instruction ([ARG1]=dest, [ARG2]=source).
-
The VARs are automatically generated temporary variables in the final code.
-
The ?+N notation indicates a relative offset to the current Program Counter location. ?+1 is a common shortcut that says continue with the next instruction regardless of the result of the operation.
-
The CLRV instruction is itself a synthetic instruction, and these can be called recursively.
-
Note the convoluted method of ensuring the destination memory address is only updated once during the instruction. A simpler method may have it hold a number of values during the transfer, which can be very problematic in a memory mapped system, where an I/O device may be monitoring a memory location for a particular value.
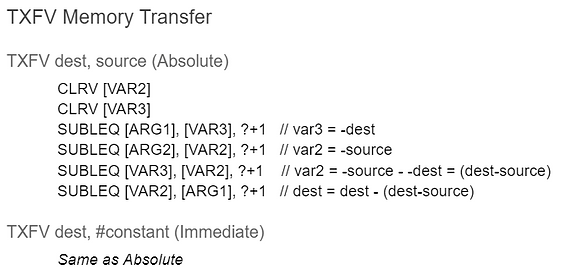
The NOT instruction is shown as an example of an instruction that includes a loop (SUBLEQ is very limited on its own) and utilises many other synthetic instructions. Some relatively low-level synthetic instructions can result in hundreds of SUBLEQ instructions after assembly (such as XOR). Keep in mind that the only test condition is that the highest bit is a 1 or that all bits are 0s.
​
The AND instruction is even more complex.
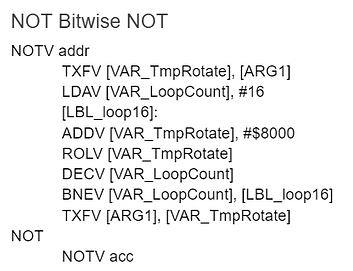
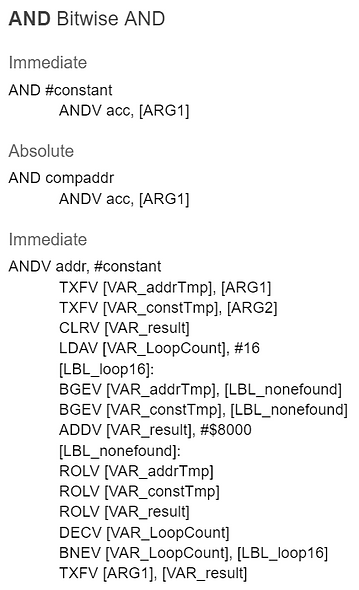
Programs
The listing below shows the general layout of a program written with synthetic instructions. The assembler checks for overlaps in the program code and allocated regions for variables and constants. This is important because the synthetic instructions can inherently generate many variables and constants, although it reuses them where possible.
​
This particular program was used to check the stack operations and the calling of functions that operate on (stack-passed) arguments and return a result (via the stack).
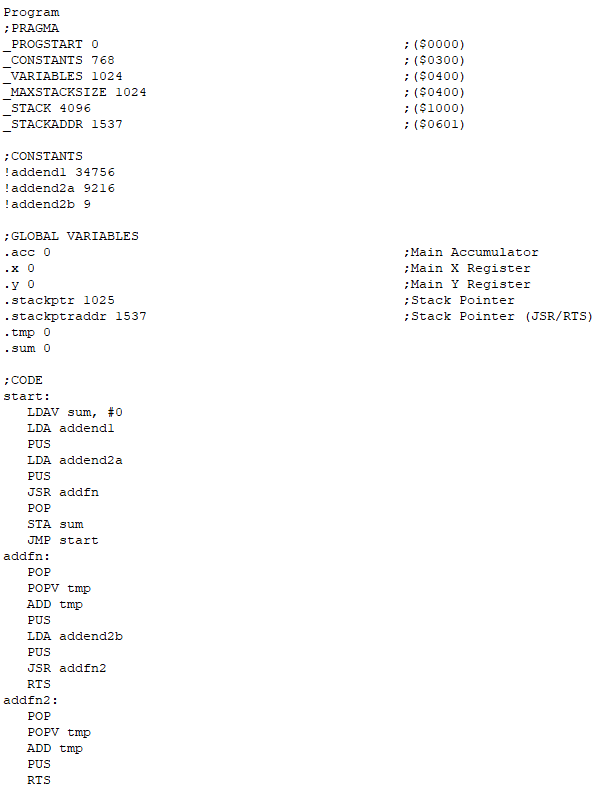
Once compiled, the program is stored in EEPROMs on the boot module. This is copied into working RAM upon system boot. Note the handy Boot Pause button that enables the copying to be done at full speed while the button is held down, and the system paused while the clock speed is reduced (or put into step-mode) for debugging purposes if required.
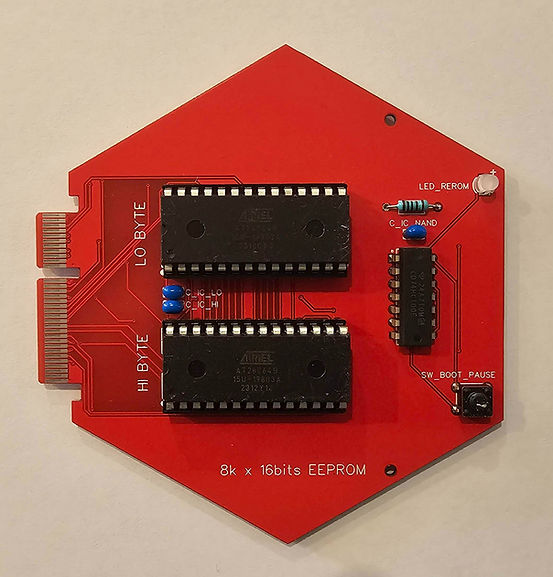
Emulator
The emulator allows a compiled hex image to be simulated, paused, and stepped through. Watches can be set for variables, virtual registers, and memory locations. Memory locations within the source code are highlighted in brackets when they have been changed by the program, and the stacks are shown with their contents. The emulator makes use of the original source code to provide a high-level view of the current synthetic instruction that is being processed, but includes the compiled low-level SUBLEQ operations for fine-grained analysis. Breakpoints can be set to pause the emulation, and the emulation run speed can be set, which is helpful in various situations.
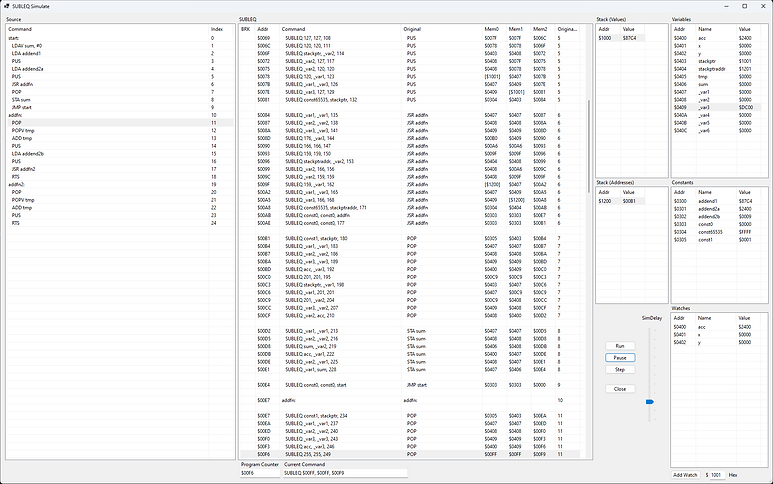